Code generation and artifacts preview with WebUI and codegemma:7b
Generate WebGL, Three.JS, HTML, CSS, JavaScript, no Python code, single page with rotating cube, ambient lighting. Load libraries from CDN. Let ambient lighting be as such cube edges are visible. add directional lighting also pointing at the cube. Scene needs to be navigable using arrow keys. Ensure browser compability.
With codegemma:7b you can generate source code. If asked properly then in WebUI chat a artifacts feature will appear, interpreting your source code immediately, just after source code is generated. This feature is useful for designers, developers and marketers who would like to speed-up scaffolding and migrating from brainstorm into visible working result.
You can use visualization libraries, Three.js, D3.js or any other HTML, CSS and JavaScript code. It is important to ask for single-page one-file content with all libraries loaded from CDN. Use words “change” or “update” to modify content or “rewrite”, “redesign” for complete rework.
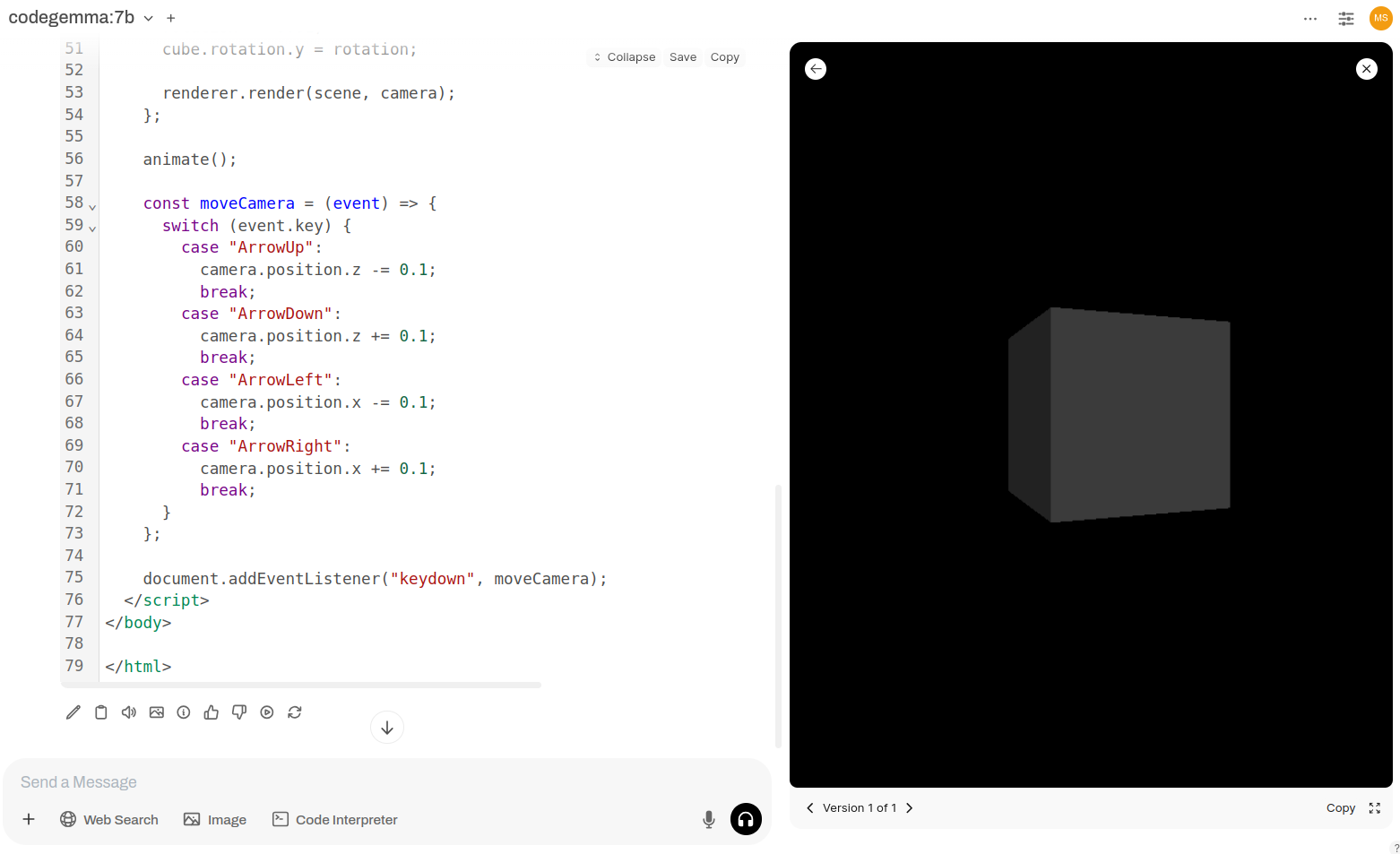
And here is the source code generated by codegemma:7b:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<title>Rotating Cube with Ambient and Directional Lighting</title>
<style>
body {
margin: 0;
overflow: hidden;
}
canvas {
display: block;
}
</style>
</head>
<body>
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r134/three.min.js"></script>
<script>
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 100);
camera.position.z = 3;
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
const geometry = new THREE.BoxGeometry(1, 1, 1);
const material = new THREE.MeshLambertMaterial({ color: 0x444444 });
const cube = new THREE.Mesh(geometry, material);
scene.add(cube);
const ambientLight = new THREE.AmbientLight(0xffffff, 0.5);
scene.add(ambientLight);
const directionalLight = new THREE.DirectionalLight(0xffffff, 0.5);
directionalLight.position.set(5, 5, 5);
scene.add(directionalLight);
let rotation = 0;
const animate = () => {
requestAnimationFrame(animate);
rotation += 0.01;
cube.rotation.y = rotation;
renderer.render(scene, camera);
};
animate();
const moveCamera = (event) => {
switch (event.key) {
case "ArrowUp":
camera.position.z -= 0.1;
break;
case "ArrowDown":
camera.position.z += 0.1;
break;
case "ArrowLeft":
camera.position.x -= 0.1;
break;
case "ArrowRight":
camera.position.x += 0.1;
break;
}
};
document.addEventListener("keydown", moveCamera);
</script>
</body>
</html>